Today we’re going to build an expression-driven arc stroke.
Start with creating any kind of shape. For example ellipse.
Now go to your shape layer and expand the contents. You can delete the Ellipse 1 path. Right next to Contents, you should see an Add option with a right arrow next to it.
Now click on this arrow and add a path.
Initially of course your path will be empty. Expand your path options to see the time-watch icon.

Now alt-click the stop-watch icon. This will add an expression to your path property.

Now delete the current expression and add the one below.
// Expression for a shape layer's Path property to create an arc as a single-stroke
// Slider controls
var startAngle = effect("Start Angle")("Slider"); // Starting angle (in degrees)
var arcAngle = effect("Angle Control")("Slider"); // Arc angle (in degrees)
var radius = effect("Radius")("Slider"); // Radius of the arc
var resolution = effect("Resolution")("Slider"); // Number of points along the arc
// Ensure resolution is at least 2
if (resolution < 2) { resolution = 2; }
var pts = []; // Array to store points on the path
var inTangents = []; // Array for in tangents (set to zero for a straightforward stroke)
var outTangents = []; // Array for out tangents (set to zero as well)
// Convert angles to radians for trigonometric functions
var startRads = degreesToRadians(startAngle);
var endRads = degreesToRadians(startAngle + arcAngle);
// Generate points along the arc
for (var i = 0; i < resolution; i++){
var alpha = i / (resolution - 1);
var theta = startRads + alpha * (endRads - startRads);
var x = radius * Math.cos(theta);
var y = radius * Math.sin(theta);
pts.push([x, y]);
// No tangents needed since we are drawing a straight stroke along calculated points
inTangents.push([0, 0]);
outTangents.push([0, 0]);
}
// Create the path from points, with no tangents, and set as open (false)
createPath(pts, inTangents, outTangents, false);
Now we need to add our expression controls as below:
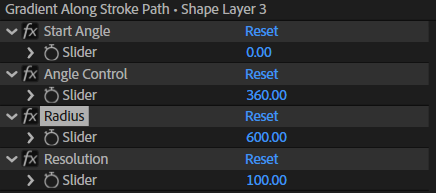
Go to Effect – Expression Controls and choose Slider.
Rename the Slider Expression Control as Start Angle.
Now Duplicate the slider control and rename it as Angle Control, then again for Radius and Resolution.
Basically, Resolution value is controlling the point amount of your arc. And done, now you have a dynamic expression controlled arc tool!
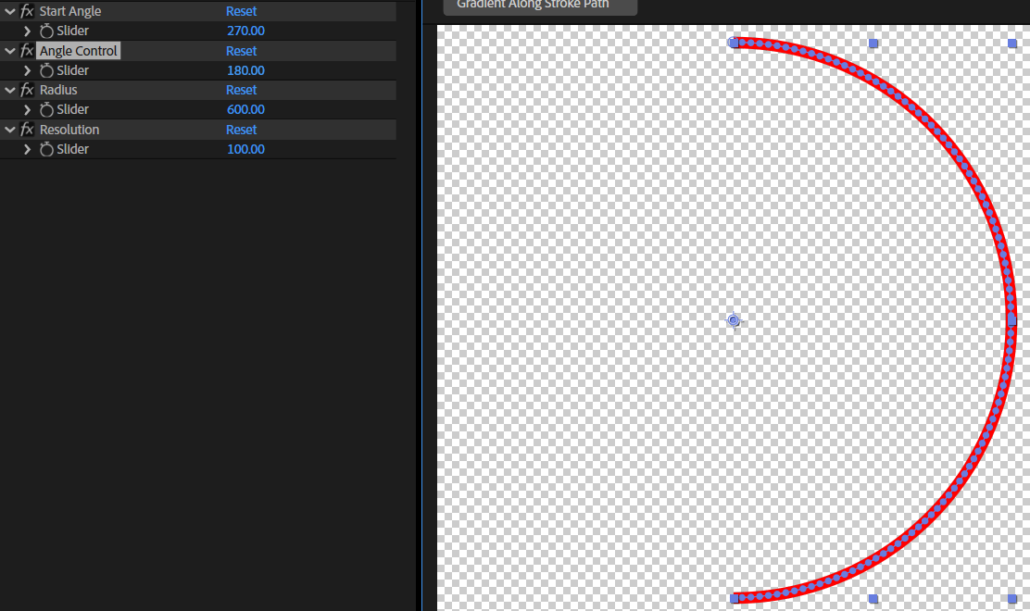