This script for Adobe Illustrator automates the duplication of a selected object along a circular path. It checks if an object is selected, prompts for the number of copies, calculates the appropriate radius based on the object’s dimensions, and then creates duplicates evenly spaced and rotated along the circle. Ideal for creating radial designs quickly and efficiently.
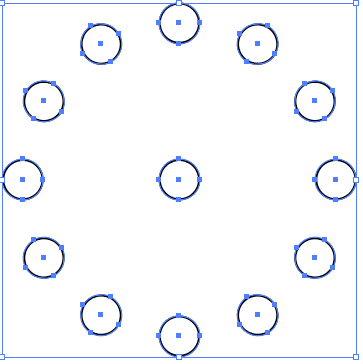
Usage:
- Open an Adobe Illustrator document and select the object you wish to duplicate.
- Run the script.
- When prompted, enter the desired number of copies.
- The script will duplicate the object and arrange the copies along a circular path with appropriate rotation.
JavaScript
//@target illustrator
function duplicateAlongCircle() {
if (app.documents.length === 0 || app.selection.length === 0) {
alert("Please select an object to duplicate.");
return;
}
var selectedObject = app.selection[0];
var numberOfCopies = parseInt(prompt("Enter the number of copies:", "6"), 10);
if (isNaN(numberOfCopies) || numberOfCopies < 1) {
alert("Please enter a valid number of copies.");
return;
}
// Calculate the radius based on the object's size
var objectWidth = selectedObject.width;
var objectHeight = selectedObject.height;
var radius = Math.max(objectWidth, objectHeight) * numberOfCopies / Math.PI;
var angleStep = 360 / numberOfCopies; // Angle in degrees between each copy
var centerX = selectedObject.position[0] + objectWidth / 2;
var centerY = selectedObject.position[1] - objectHeight / 2;
for (var i = 0; i < numberOfCopies; i++) {
var angle = i * angleStep * (Math.PI / 180); // Convert to radians
var x = centerX + radius * Math.cos(angle) - objectWidth / 2;
var y = centerY + radius * Math.sin(angle) + objectHeight / 2;
var duplicate = selectedObject.duplicate();
duplicate.position = [x, y];
duplicate.rotate(angleStep * i);
}
alert(numberOfCopies + " copies created along a circular path.");
}
duplicateAlongCircle();