Noise Jitter Cinema 4D Python Effector
The Noise Jitter Effector is a versatile and easy-to-use tool designed for motion designers using Cinema 4D. This effector introduces a subtle, randomized offset to the position of MoGraph elements, creating a dynamic jittering effect that evolves over time. It’s particularly useful for adding natural-looking motion and imperfections to your animations, making your scenes feel more organic and lively.
How to Use:
- Add the Effector: Insert the Noise Jitter Effector into your MoGraph setup.
- Automatic Randomization: The effector uses a fixed random seed for consistent noise patterns, ensuring predictable and repeatable results across renders.
- Time-Based Variation: The jitter effect dynamically changes over time, modulated by the current frame to create smooth, evolving randomness.
- Customization:
- Adjust the maximum jitter strength by modifying the
max_offset
value in the script. - Use the built-in randomness to simulate natural movement with minimal setup.
This effector is ideal for creating subtle movements, such as shaking, flickering, or adding imperfections to elements in your motion graphics. Whether you’re animating text, particles, or abstract geometries, the Noise Jitter Effector makes it effortless to add dynamic energy to your scenes.
Python
"""
NoiseJitterEffector.py
Creates a jitter effect for a MoGraph object by applying a subtle random offset
to each element's position. The random seed is set to ensure consistent noise
patterns, and the effect varies based on the current frame.
"""
import c4d, random, math
# Set a fixed random seed to maintain consistency in the jitter effect
random.seed(5678)
def main() -> bool:
# Get the MoGraph data
data = c4d.modules.mograph.GeGetMoData(op)
if data is None:
return True
# Get current frame (can be used to vary the jitter over time)
frame = doc.GetTime().GetFrame(doc.GetFps())
matrices = data.GetArray(c4d.MODATA_MATRIX)
count = data.GetCount()
# Define the maximum jitter strength
max_offset = 50.0
for i in range(count):
matrix = matrices[i]
pos = matrix.off
# Apply a random factor modulated by frame to simulate jitter
jitter_x = random.uniform(-max_offset, max_offset) * (0.5 + 0.5 * math.sin(frame * 0.1))
jitter_y = random.uniform(-max_offset, max_offset) * (0.5 + 0.5 * math.cos(frame * 0.1))
jitter_z = random.uniform(-max_offset, max_offset) * (0.5 + 0.5 * math.sin(frame * 0.1))
# Update the element's position with jitter
matrix.off = c4d.Vector(pos.x + jitter_x, pos.y + jitter_y, pos.z + jitter_z)
matrices[i] = matrix
# Apply the new matrices
data.SetArray(c4d.MODATA_MATRIX, matrices, op[c4d.FIELDS].HasContent())
return True
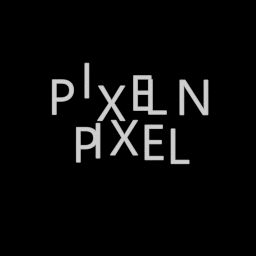