Random Layer Order Script
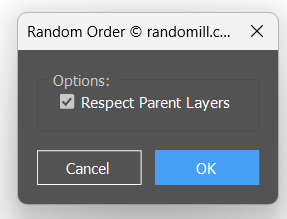
This Adobe Illustrator script randomizes the stacking (z-order) of the selected objects. It is a stripped-down version of the Random Layer Order functionality originally part of the Randomill Illustrator Plugin. The script was created by Boris Boguslavsky in 2022 and is available on GitHub.
Before script:
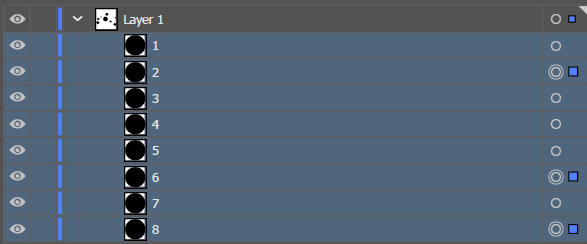
After script:
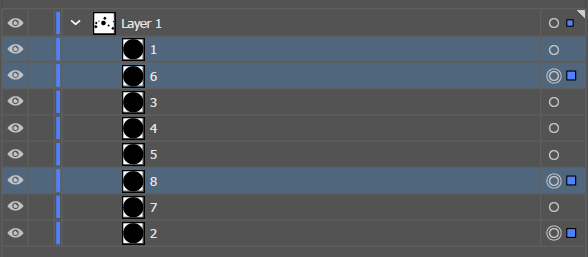
Purpose
- Randomize Layer Order:
The script rearranges the order of objects (layers) randomly. This can be useful for creative effects or for quickly shuffling the order of items in your document. - Respect Parent Layers (Optional):
An option in the user interface lets you choose whether to randomize the order while respecting the original parent layer hierarchy. When enabled, the objects are grouped by their parent layer, and randomization is performed within each group.
Description
- User Interface:
When you run the script, a dialog window is displayed. This window includes: - An options panel with a checkbox labeled “Respect Parent Layers”. When checked, objects are shuffled only within their respective parent layers.
- “OK” and “Cancel” buttons for executing or aborting the process.
- Input Requirements:
The script requires that you have at least two objects selected in your document. If there are fewer than two or if they are grouped improperly, the script will display an error message. - Core Functionality:
The script first determines the selected objects. Depending on the user’s choice regarding parent layers: - If the “Respect Parent Layers” option is enabled, it groups the selected objects by their parent layer and shuffles each group independently.
- If disabled, the script shuffles the entire selection. Under the hood, the script uses a Fischer-Yates shuffle to randomize an array of indices corresponding to the selected objects. It then swaps the stacking order (z-order) by changing the
.selected
flag of objects and using a temporary duplicate object as needed to perform the swap. - Error Handling:
The script checks for the presence of an open document and verifies that a valid selection (with at least two items) has been made. If these conditions are not met, it prompts the user with an error message.
Usage Instructions
- Open an Adobe Illustrator Document:
Ensure that you have a document open which contains at least two objects (paths, shapes, etc.). - Make a Selection:
Select the objects that you want to randomize. For more accurate behavior, especially when using the parent layers option, ensure that objects are not overly grouped if you want finer control. - Run the Script:
Execute the script (for example, viaFile > Scripts
in Illustrator). The dialog titled “Random Order © randomill.com” will appear. - Set Your Options:
- Respect Parent Layers:
Check this option if you want the randomization to happen within each parent layer separately. Leave it unchecked if you want to completely randomize the order regardless of parent grouping.
- Confirm or Cancel:
Click “OK” to initiate the randomization process. If you change your mind, click “Cancel” to exit without making any changes. - View the Results:
The selected objects will have their layer order randomized based on your chosen options.
Feel free to modify the script to best suit your workflow. Enjoy the creative possibilities!
JavaScript
Random Layer Order – Illustrator Script (0 downloads )
var mainPanelWindow = new Window('dialog', 'Random Order \u00A9 randomill.com', undefined);
mainPanelWindow.orientation = 'column';
mainPanelWindow.alignChildren = ['fill', 'fill'];
var optionsGroup = mainPanelWindow.add('panel', undefined, 'Options:');
optionsGroup.orientation = 'column';
optionsGroup.alignChildren = ['fill', 'fill'];
var respectParentLayers = optionsGroup.add('checkbox', undefined, 'Respect Parent Layers');
respectParentLayers.value = true;
var cancelAndOkButtons = mainPanelWindow.add('group');
cancelAndOkButtons.alignChildren = ['fill', 'fill'];
cancelAndOkButtons.margins = [0, 0, 0, 0];
var cancel = cancelAndOkButtons.add('button', undefined, 'Cancel');
cancel.helpTip = 'Press Esc to Close';
cancel.onClick = function () {
mainPanelWindow.close();
}
var ok = cancelAndOkButtons.add('button', undefined, 'OK');
ok.helpTip = 'Press Enter to Run';
ok.onClick = function () {
try {
randomizeLayerOrder();
} catch(err) {
alert(err)
}
mainPanelWindow.close();
}
ok.active = true;
function randomizeLayerOrder(objects) {
if (app.documents.length === 0) {
alert("Error: No currently active document.");
return;
}
var objects = app.activeDocument.selection;
if (objects.length < 2) {
alert("Error: Please select at least two items. If more than two items are selected, make sure they're ungrouped.");
return;
}
if (respectParentLayers.value) {
shuffleLayersRespectParents(objects)
} else {
shuffleLayers(objects)
}
return;
}
function shuffleLayers(objects) {
var shuffled = shuffleArray(objects)
var loopLength = objects.length%2===0 ? objects.length / 2 : Math.floor(objects.length / 2) + 1
for (var i=0; i < loopLength; i++) {
var a = shuffled[i*2]
var b = shuffled[(i*2)+1]
if (!b) {
b = shuffled[0]
}
swapTwoLayers(a, b)
}
}
function shuffleLayersRespectParents(objects) {
if (objects.length < 2) return;
var separatedObjects = collectObjectsByParentLayers(objects);
var separatedParentLayers = getKeys(separatedObjects)
for (var i=0; i < separatedParentLayers.length; i++) {
shuffleLayers(separatedObjects[separatedParentLayers[i]])
};
}
function swapTwoLayers(a, b) {
var temp = b.duplicate(b)
b.move(a, ElementPlacement.PLACEBEFORE)
a.move(temp, ElementPlacement.PLACEAFTER)
temp.remove()
}
function collectObjectsByParentLayers(objects) {
var objects = app.activeDocument.selection;
var topArr = new Object();
for (var i=0; i < objects.length; i++) {
if (!topArr[objects[i].layer.absoluteZOrderPosition]) topArr[objects[i].layer.absoluteZOrderPosition] = new Array();
topArr[objects[i].layer.absoluteZOrderPosition].push(objects[i])
}
return topArr;
}
function getKeys(obj) {
if (Object(obj) !== obj) {
throw new TypeError('Object.keys can only be called on Objects.');
}
var hasOwnProperty = Object.prototype.hasOwnProperty;
var result = [];
for (var prop in obj) {
if (hasOwnProperty.call(obj, prop)) {
result.push(prop);
}
}
return result;
};
function shuffleArray(array) {
var curId = array.length;
while (0 !== curId) {
var randId = Math.floor(Math.random() * curId);
curId -= 1;
var tmp = array[curId];
array[curId] = array[randId];
array[randId] = tmp;
}
return array;
}
mainPanelWindow.center();
mainPanelWindow.show();