Exploring the Self-Contained Magnetic Swarm Effector
In this article, we delve into a fascinating Python script that simulates a magnetic swarm effector. This self-contained script leverages basic randomness to introduce turbulence, offering a simplified yet powerful approach to simulating swarm behavior without relying on external dependencies. Whether you’re into motion graphics, physics simulations, or creative coding, this article will walk you through the script’s functionality, available options, and potential use cases.
Overview
The script, titled Self-Contained Magnetic Swarm Effector, is designed to generate a dynamic, interactive swarm simulation. It features:
- No external dependencies: Utilizes Python’s built-in libraries such as
random
andmath
for noise and turbulence. - Simplified noise generation: Implements a custom pseudo-noise function called
simple_noise
to mimic the behavior of Perlin noise. - Flocking behavior: Incorporates basic principles of flocking, including cohesion, alignment, and magnetic separation forces.
- Goal seeking: Allows the swarm to be directed toward a target goal position.
- Turbulence: Applies additional randomness to simulate natural, unpredictable movement.
Key Components
1. Pseudo-Noise Generation
The simple_noise
function replaces more complex external noise libraries by seeding Python’s random module with a combination of coordinate data. It returns a value between -1 and 1, simulating a noise function that can be used to perturb the swarm’s movements:
def simple_noise(x, y, z, seed):
"""Built-in pseudo-noise function to replace Perlin noise"""
random.seed(int(x*100 + y*1000 + z*10000 + seed))
return random.random() * 2 - 1 # Returns -1 to 1
2. Swarm Initialization and Storage
The script uses a global dictionary called swarm_system
to keep track of positions, velocities, accelerations, and random seeds. This allows it to maintain state between frames and efficiently update the swarm’s behavior.
3. Flocking and Magnetic Interactions
Within the main
function, the script calculates forces for each particle based on nearby neighbors:
- Cohesion: Agents are drawn toward the average position of their neighbors.
- Alignment: Agents try to match the average velocity of surrounding agents.
- Magnetic Behavior: Agents repel each other to prevent overcrowding, with the repulsive force inversely related to distance.
This behavior creates a balance between unity (flocking) and separation (magnetic repulsion), resulting in a natural-looking swarm movement.
4. Goal Seeking and Turbulence
An optional goal object can be defined, driving the swarm toward a specified target. Additionally, turbulence is added to each agent’s acceleration using the simplified noise function, giving the simulation a more organic, unpredictable feel.
5. User Parameters
The script supports various user-defined parameters, configurable via a user-data interface:
- Flock Radius: Defines the area in which an agent considers its neighbors.
- Cohesion and Alignment: Scale factors determining the strength of flocking forces.
- Magnetism: Controls the repulsive force between agents.
- Goal Strength: Determines how strongly agents are attracted to the goal.
- Turbulence: Adds randomness to simulate environmental disturbances.
- Max Speed: Limits how fast an agent can move.
- Debug Visualization: Optionally displays force vectors for in-depth debugging.
Potential Usage
This script can be particularly useful for:
- Motion Graphics and Animation: Creating dynamic swarm animations for background effects or artistic visuals.
- Simulation and Game Development: Implementing simple flocking behavior to simulate crowds, fish schools, or bird flocks.
- Educational Purposes: Demonstrating principles of physics, vector math, and procedural noise generation.
- Interactive Installations: Integrating with graphics software like Cinema 4D for real-time visual effects.
How It Works
- Initialization:
- Agents’ positions, velocities, and acceleration vectors are initialized based on the current state of the scene.
- Random seeds are generated to provide unique noise values for turbulence.
- Force Calculation:
- For each frame, the script calculates the contribution of cohesion, alignment, magnetic repulsion, goal seeking, and turbulence to update each agent’s velocity and position.
- Visualization:
- Optionally, the script can render debug lines to show the forces acting on each agent, which is valuable for fine-tuning and debugging the simulation.
- Update Cycle:
- The agents’ new positions are written back to the scene data, updating the visual representation in real time.
Conclusion
The Self-Contained Magnetic Swarm Effector is a compact, robust script that packs a range of features into a neat package. Its design exemplifies how simple algorithms and basic randomness can be combined to produce complex, lifelike behaviors. Whether you’re tweaking parameters for a specific visual effect or exploring the fundamentals of swarm behavior, this script is an excellent tool for creative coding and simulation in motion graphics.
Happy coding, and may your swarms always behave magnetically!
"""
Self-Contained Magnetic Swarm Effector
No external dependencies - uses built-in random for turbulence
Same features as original but with simplified noise
"""
import c4d
import math
import random
# Global swarm storage
swarm_system = {
"positions": [],
"velocities": [],
"accelerations": [],
"initialized": False,
"random_seeds": []
}
def simple_noise(x, y, z, seed):
"""Built-in pseudo-noise function to replace Perlin noise"""
random.seed(int(x*100 + y*1000 + z*10000 + seed))
return random.random() * 2 - 1 # Returns -1 to 1
def main() -> bool:
global swarm_system
# Get MoGraph data
data = c4d.modules.mograph.GeGetMoData(op)
if data is None:
return True
# Get current frame and count
frame = doc.GetTime().GetFrame(doc.GetFps())
matrices = data.GetArray(c4d.MODATA_MATRIX)
count = data.GetCount()
# Access user data
flock_radius = op[c4d.ID_USERDATA, 1] or 150.0
cohesion = op[c4d.ID_USERDATA, 2] or 0.8
alignment = op[c4d.ID_USERDATA, 3] or 1.2
magnetism = op[c4d.ID_USERDATA, 4] or 3.0
goal_strength = op[c4d.ID_USERDATA, 5] or 1.5
turbulence = op[c4d.ID_USERDATA, 6] or 0.3
max_speed = op[c4d.ID_USERDATA, 7] or 150.0
goal_object = op[c4d.ID_USERDATA, 8]
show_forces = op[c4d.ID_USERDATA, 10] or False
# Handle reset button
reset_simulation = False
button_state = op.GetDataInstance().GetContainer(c4d.ID_USERDATA).GetData(c4d.ID_USERDATA + 9)
if button_state and button_state.GetInt32(c4d.BITBUTTON_CLICKED):
reset_simulation = True
button_state.SetInt32(c4d.BITBUTTON_CLICKED, 0)
op.SetDirty(c4d.DIRTYFLAGS_DATA)
# Initialize system
if not swarm_system["initialized"] or reset_simulation or len(swarm_system["positions"]) != count:
swarm_system["positions"] = [m.off for m in matrices]
swarm_system["velocities"] = [c4d.Vector(
random.random()-0.5,
random.random()-0.5,
random.random()-0.5
) * 10 for _ in range(count)]
swarm_system["accelerations"] = [c4d.Vector(0,0,0) for _ in range(count)]
swarm_system["random_seeds"] = [random.randint(0,1000) for _ in range(count)]
swarm_system["initialized"] = True
try:
# Get goal position
goal_pos = goal_object.GetMg().off if goal_object else c4d.Vector(0,0,0)
for i in range(count):
pos = swarm_system["positions"][i]
vel = swarm_system["velocities"][i]
accel = c4d.Vector(0,0,0)
seed = swarm_system["random_seeds"][i]
# FLOCKING BEHAVIOR
neighbors = []
center = c4d.Vector(0,0,0)
avg_vel = c4d.Vector(0,0,0)
neighbor_count = 0
for j in range(count):
if i == j:
continue
dist = (pos - swarm_system["positions"][j]).GetLength()
if dist < flock_radius:
neighbors.append(j)
center += swarm_system["positions"][j]
avg_vel += swarm_system["velocities"][j]
neighbor_count += 1
if neighbor_count > 0:
center /= neighbor_count
avg_vel /= neighbor_count
accel += (center - pos).GetNormalized() * cohesion
accel += avg_vel.GetNormalized() * alignment
# MAGNETIC BEHAVIOR
if abs(magnetism) > 0.1:
for j in neighbors:
delta = pos - swarm_system["positions"][j]
dist = delta.GetLength()
if dist > 0:
force = delta.GetNormalized() * magnetism / (dist * 0.1)
accel += force
# GOAL SEEKING
if goal_strength > 0:
to_goal = (goal_pos - pos).GetNormalized()
accel += to_goal * goal_strength
# SIMPLIFIED TURBULENCE
if turbulence > 0:
time = frame * 0.05
accel.x += simple_noise(pos.x*0.1, pos.y*0.1, time, seed) * turbulence
accel.y += simple_noise(pos.y*0.1, time, pos.z*0.1, seed+1) * turbulence
accel.z += simple_noise(time, pos.z*0.1, pos.x*0.1, seed+2) * turbulence
# Update physics
vel += accel
speed = vel.GetLength()
if speed > max_speed:
vel = vel.GetNormalized() * max_speed
swarm_system["velocities"][i] = vel
swarm_system["positions"][i] += vel * 0.1
matrices[i].off = swarm_system["positions"][i]
# Orientation
if speed > 0.1:
forward = vel.GetNormalized()
up = c4d.Vector(0,1,0) if abs(forward.y) < 0.99 else c4d.Vector(0,0,1)
right = up.Cross(forward).GetNormalized()
up = forward.Cross(right).GetNormalized()
matrices[i].v1 = right
matrices[i].v2 = up
matrices[i].v3 = forward
# Debug visualization
if show_forces:
c4d.utils.DrawLine(pos, pos+accel*20, c4d.Vector(1,0,0), c4d.DRAWLINE_FORCEFULL)
c4d.utils.DrawLine(pos, pos+vel, c4d.Vector(0,1,0), c4d.DRAWLINE_FORCEFULL)
if goal_object:
c4d.utils.DrawLine(pos, goal_pos, c4d.Vector(1,1,0), c4d.DRAWLINE_FORCEFULL)
except Exception as e:
print(f"Swarm Error: {str(e)}")
swarm_system["initialized"] = False
return True
data.SetArray(c4d.MODATA_MATRIX, matrices, op[c4d.FIELDS].HasContent())
return True
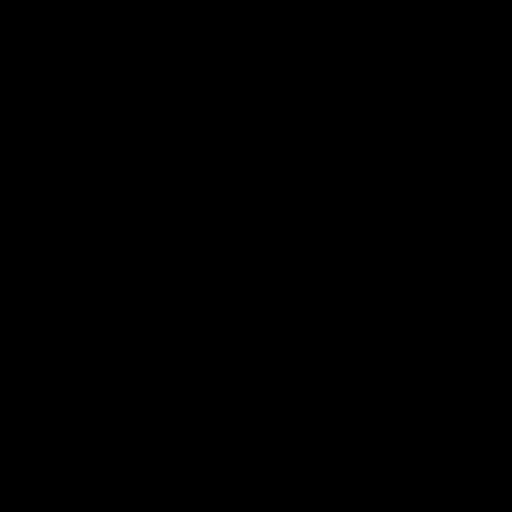